Enumerable#group_by | My new favorite Enumerable method
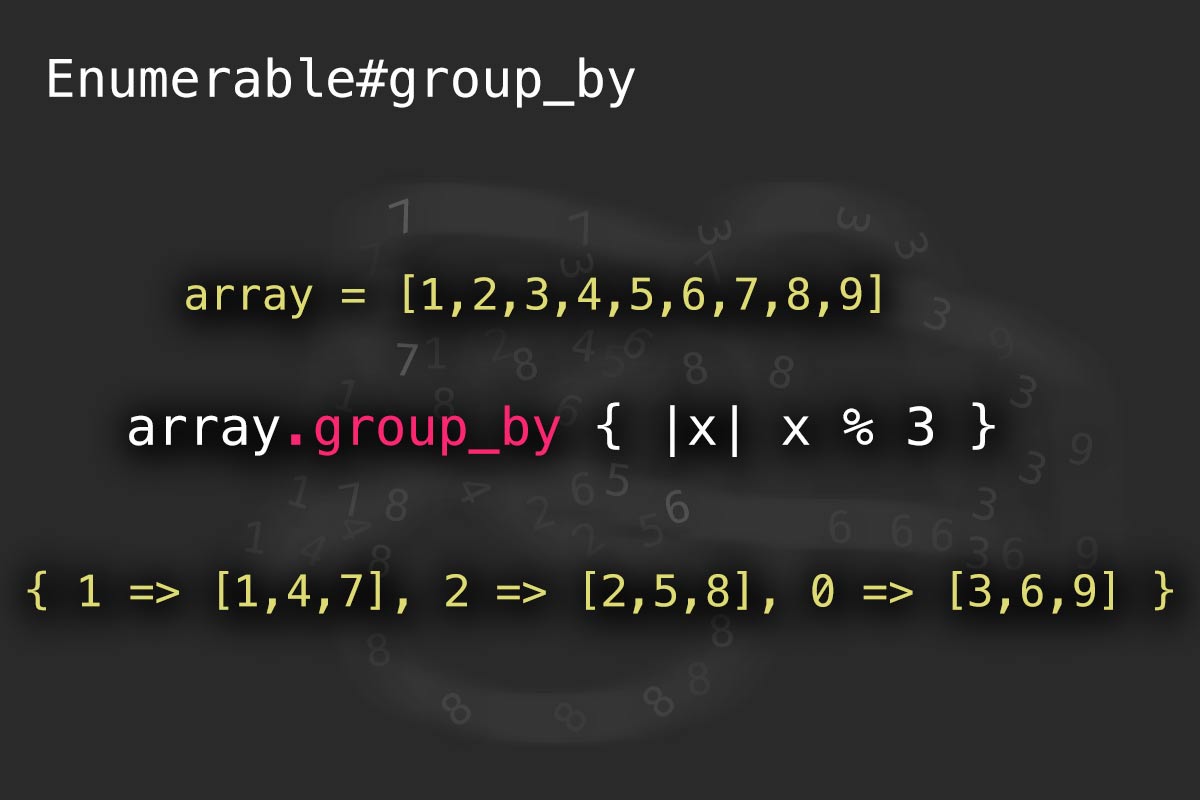
Enumerable#group_by takes an Array and groups it by an argument
If you've never used Enumerable#group_by you might not be aware of this useful grouping tool
Chances are you've skimmed past this in the Ruby.org docs and didn't give it a second thought. It comes right after the ever popular Enumerable#grep method and it only takes up a few lines. The docs, however well written, just don't do this little method justice.
Let's take a look at what makes it so special and then we'll walk through a real world example to see how we might put this to use.
#group_by is a method that can iterate over an Array or a Hash and, as the name implies, group the elements into a new Hash with keys representative of the evaluated result from the code block used to generate it. Seems simple enough right? So let's see how it works:
In the example below, we have an Array of names called 'array' which we call #group_by on using the length of each name as the evaluator. We end up with a hash stored in 'result' which we then use #sort_by on to get a ranking of names by largest first:
group_by.rb -- your-project.com
result = array.group_by { |name| name.length }
puts (result.sort_by { |k,v| k }).reverse.inspect
# Output:
# [[11, ["Christopher"]], [9, ["Alexander", "Christian"]], [8, ["Danielle"]], [5, ["Abbie"]], [4, ["Ajay", "Alex"]]]
Line yy, Column xx
Spaces: 4 RUBY
In the next line, we use #sort_by on the result hash then #reverse and #inspect. The #sort_by method turns our output back into an Array, but the key, value pairs still exist. We could easily turn this back into a hash if necessary.
Note that the keys in the key value pair are the #length of each word as we called in the #group_by method.
If you haven't done so yet, pull up an IRB session or just create a new .rb file and play around with Enumereable#group_by for awhile. There's bound to be a moment when you find a perfect use for this, and you'll be glad you took the time to get to know it.
Let's do something EPIC!