A Class for Dev Bootcamp Boots
Yah. I did it. Oh and it's my birthday!!!! @devbootcamp pic.twitter.com/IZNV74E5Nm
— Lorena Mesa (@loooorenanicole) March 7, 2014
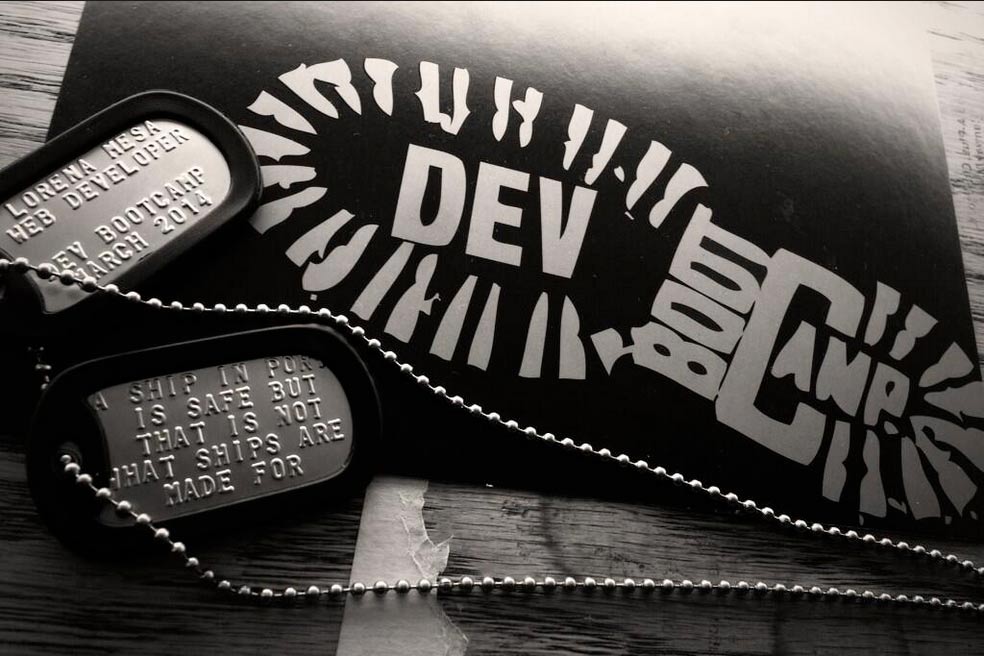
Dev Bootcamp Boots deserve a Class to model their pursuit of DBC Dogtags
Class is an important concept in Ruby. As Boots are important at DBC, it's only fitting that they should have their own Ruby Class.
Class is one of the most important concepts in the Ruby languange. Classes allow us to define objects. We instantiate an object in Ruby by calling [Class Name].new and when we do so, we have created a new object with the Class of whatever we named it. We can even alter existing Classes in Ruby by opening and defining them again.
We can create a Class to model real world objects. If you are writing a program for something, you will most likely pick at least one real world element to model with a Class, and in most instances, you will model more than one with a Class.
To demonstrate this, I thought it would be fun and helpful to model a real world object that is very near and dear to my heart. The Boot is also a very important part of the Dev Bootcamp system. One could certainly argue that it is the most important part.
So, how do we know when to use a Class to model an object? Well, anytime that we have an object that needs to be manipulated and have it's own characteristics or methods, then we need to create a Class for it.
Take a look at the Boot Class below and I think it will start to make more sense. Keep in mind that I have added quite a bit of non-essential code to this. It's kind of a Rube Goldberg edition. I think if you copy and paste this into a new .rb document and play with it for awhile, it might help you to understand classes and how they work.
boot_class.rb -- DevBootcamp.com
class Boot
def initialize(name, start_date)
@name = name
@start_date = start_date
@dogtags = false
@this_day = nil
@this_week = week
unless @dogtags == true
puts "\nThis is week ##{@this_week} and this is day ##{@this_day}"
puts "#{@name} will receive his/her Dev Bootcamp Dogtags in #{126 - @this_day} days"
puts "\nWould you like to see #{@name}'s schedule for today?"
choice = gets.chomp
day if choice.downcase == "yes"
end
end
def day
puts
eat("Breakfast", 0.75)
code(4)
eat("Lunch", 1.25)
code(5)
eat("Supper", 1.0)
code(5)
sleep(7)
end
def sleep_faster
puts "\nHow many hours does #{@name} like to sleep each night? "
preferred_sleep_hours = gets.chomp
if preferred_sleep_hours.to_i > 7
puts "\n\"My advice would be to sleep faster\" - Arnold Schwarzenegger"
else
puts "#{@name} will get plenty of sleep at Dev Bootcamp"
end
end
def sleep(hours)
sleep_faster if hours < 8
puts "\n7.0 hr: Sleep\n\n"
end
def eat(meal, time)
puts "#{time} hr: #{meal}"
end
def code(hours)
hours.times { puts "1.0 hr: Coding..." }
end
def week
@this_day = (DateTime.now - @start_date).to_i
this_week = ((DateTime.now - @start_date).ceil.to_i / 7)
if this_week > 18
@dogtags = true
get_your_dogtags
end
this_week
end
def get_your_dogtags
puts "\nHas #{@name} completed all of their work at Dev Bootcamp? "
completion = gets.chomp.downcase
if completion == "yes" && @dogtags == true
puts "\n\nYay! #{@name} has earned his/her Dogtags!\n\n\n"
else
puts "Sorry, #{@name} has not met all of the requirements to receive his/her Dogtags yet."
end
end
end
puts "Name: "
name = gets.chomp
puts "Phase 0 Start Date (MM/DD/YYYY): "
date = gets.chomp
date = date.split("/")
month = date[0].to_i
day = date[1].to_i
year = date[2].to_i
start_date = DateTime.new(year,month,day,12,0)
boot = Boot.new(name, start_date)
puts boot.get_your_dogtags
Line yy, Column xx
Spaces: 4 RUBY
We start off with the 'initialize' method. This is a special method that runs automatically when an object of this class is instantiated. So, when we call 'Boot.new(name, start_date)' we are instantiating a new object with a Class of Boot. Everything in the 'initialize' method is run immediately including any methods which are called inside the 'initialize' method.
Any of the methods defined inside the Class are also available to be called on the object outside of the class. I think you can see now why the ability to create Classes is so powerful. They allow us to add a great deal of complexity to a program with a very simple call.
On the last line in this code snippet, we call 'get_your_dogtags' from outside the class, on our 'boot' variable which is holding an instance of the 'Boot' Class. As we already have an instance of the class, we can very easily access this method with dot notation and find out if, in fact, our current boot has earned their dogtags.
Use of the instance variable is very important in a Class. If you define a local variable inside a method, it will not be available in the rest of the Class. In order to be able to access a variable anywhere in the Class you must designate it as such with an '@' symbol as the first character.
Any arguments which are passed in during instantiation are passed to the 'initialize' method and it is typical to initialize new instance variables with the same name as the local argument variable. This is good practice and ensures that these variables will be accessible in the entire class. It is also possible to initialize instance variables outside of the 'initialize' method.
The more you use Classes, the more you will understand them. If you are still struggling to wrap your head around this, try to pick a real world object and start replacing elements of this Class with whatever object you want to model. With a little experimentation, you should start to understand how and why Class is so powerful and important in the Ruby language.
Let's do something EPIC!